What's new in Angular 7

What's new in Angular v7



Gerard Sans
@gerardsans
Gerard Sans
@gerardsans
SANS
GERARD
Google Developer Expert

Google Developer Expert
International Speaker

Spoken at 109 events in 27 countries

Blogger
Blogger
Community Leader

900
1.6K
Trainer

Master of Ceremonies

Master of Ceremonies







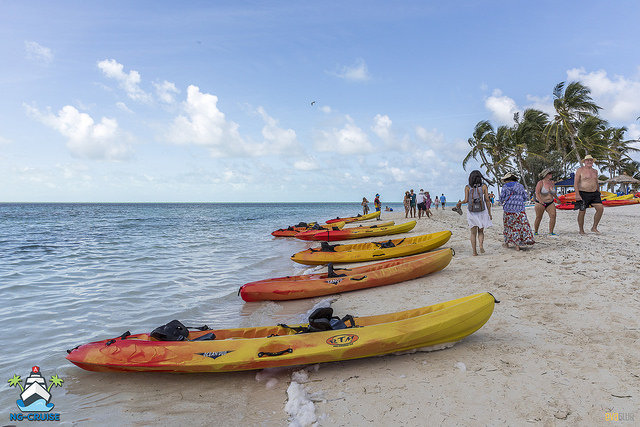




#cfpwomen


Timeline
Angular v2
Future ready
2016
Angular v4
Stable and performant
4.2 Animations
New angular.io website
4.3 HttpClient
2017
Angular v5
Smaller, Faster and Easier to use
PWA support
SSR. Material components
Bazel. Build time tools
2017
Angular v6
Smaller, Faster and Easier to use
Webpack 4. TypeScript 2.7. RxJS 6
Tooling improvements
2018
Angular v7
Smaller, Faster and Easier to use
TypeScript 3.1. RxJS 6.3
Angular Elements. Ivy Renderer
Tooling improvements
2018
Angular v7

New Versions
- Angular v7
- Angular CLI v7
- Angular Material + CDK v7
Semantic Versioning
Semantic Versioning
X . Y . Z
MAJOR MINOR PATCH
Semantic Versioning
- v2 Sept 2016
- v4 March 2017
- v5 November 2017
- v6 May 2018
- v7 October 2018
Long Term Support (LTS)
- Only critical fixes and security patches
- Angular v6 LTS begins with v7
Long Term Support (LTS)
6 months
v7
v8
v9
v10
v11
LTS (12 mo)
Goals
- Fixed release calendar
- Incremental upgrades
- Stability
- Reliability
Upgrade

update.angular.io
Breaking Changes

Base Dependencies
-
Angular Core
- TypeScript 3.1
- RxJS v6.3
-
Server Side Rendering
- Domino v2.1
- Added support Node v10
RxJS v6.3
New Features
- New operator: endWith
- New helper: isObservable
- Added index to delayWhen selector
- Added subscription to expectObservable
- Performance improvements
import { startWith, endWith } from 'rxjs/operators';
import { empty, never, of, from } from 'rxjs';
from([1,2,3]).pipe(endWith('🏄')).subscribe(log);
of(1).pipe(endWith('🏖')).subscribe(log);
empty().pipe(endWith('🚤','🌊🌊🌊')).subscribe(log);
never().pipe(endWith('🐒')).subscribe(log);
app/my.component.ts
Operator endWith
import { Observable, isObservable } from 'rxjs';
const rxjs6 = new Observable();
const rxjs5 = { lift(){}, subscribe(){} };
const other = { subscribe(){} };
console.log('RxJS 6:', isObservable(rxjs6)); // true
console.log('RxJS 5:', isObservable(rxjs5)); // true
console.log('Other', isObservable(other)); // false
app/my.component.ts
Helper isObservable
TypeScript v3.1
New Features
- Mapped object types now work on tuples and arrays (inc. Partial, Required)
- Property declarations on functions are now supported
- Specify types version using typesVersions
type MapToPromise<T> = {[K in keyof T]: Promise<T[K]>};
type Coordinate = [number, number];
type PromiseCoordinate = MapToPromise<Coordinate>;
// [Promise<number>, Promise<number>]
app/my.component.ts
Mapped Types on tuples and arrays
function memoize(fn) {
let cache, $args;
function m() {
...
}
m.clear = () => {
cache = {};
$args = [];
};
return m;
};
app/my.component.ts
Property declarations on Functions
{
"name": "package-name",
"version": "1.0",
"types": "./index.d.ts", // fallback
"typesVersions": {
">=3.1": { "*": ["ts3.1/*"] } // matches in order
}
}
// node_modules/package-name/ts3.1/index.d.ts [v3.1]
// node_modules/package-name/index.d.ts [other]
package.json
Specify TypeScript Version
Angular CLI

Angular CLI prompts
Angular CLI 7.1
-
Configurable prompts via schematics
-
ng new myProject
-
ng add @angular/material
-
-
Friendly upgrades
-
ng update @angular/cli @angular/core
-
Angular CLI 7.1
-
Size Budgets defined by default
- bundle, initial, allScript, all, anyScript, any
-
baseline, max, min, warning, error
-
bytes, KB, MB, %
-
Warning set at 2MB
-
Error set at 5MB
{
"configurations": {
"production": {
budgets: [
{ "type": "initial",
"maximumWarning":"2mb", "maximumError":"5mb"},
{ "type":"bundle", "name":"vendor",
"baseline":"500kb", "warning":"200kb", "error":"300kb" }
]
}
}
}
angular.json
Configure Size Budgets
Schematics
Overview
-
Automation Technology by Angular CLI
-
Targets repetitive actions
-
Scaffolds using Best Practices
-
Create custom templates
-
-
Applies file transforms to your project
-
Applies code transforms via ASTs
-
Extensible
"routing": {
"type": "boolean",
"description": "Generates a routing module.",
"default": false,
"x-prompt": "Would you like to add Angular routing?"
},
schematic.json
New CLI prompts
Extensible
-
Angular CLI
-
@schematics/angular
-
-
Angular Material
-
@angular/material
-
-
State Management
-
ngrx/schematics
-
ngrx/schematics
- Scaffolding templates for ngrx
-
Provides commands for
- Setting initial Store and Effects
- Actions, Reducers, Entities, Features
- Containers (injected Store)
- Automates creation and registration
ng new ngrx-entity-todo
npm install @ngrx/schematics --save-dev
npm install
@ngrx/{store, effects, entity, store-devtools} --save
Creating an App using ngrx
# Setup Default Collection
ng config cli.defaultCollection @ngrx/schematics
ng generate store State --root --module app.module.ts
ng generate effect App --root --module app.module.ts
ng generate entity Todo -m app.module.ts
Using Schematics to Setup ngrx
Angular Material

material.angular.io
Overview
-
Material Design Components for Angular
- ng add @angular/material
- Available for Web, Mobile and Desktop
- Consistent across browsers
- Flexible: theme, i18, accessibility support
Angular Material
- Form controls (input, select, checkbox, date picker and sliders),
- Navigation (menus, sidenav and toolbar)
- Layout (grids, cards, tabs and lists )
- Buttons
- Indicators (progress bars and spinners)
- Overlays (popups and modals)
- Data tables

New Material Design Specs

material.angular.io/cdk
Overview
-
Component Developer Kit
- ng add @angular/cdk
-
Provides common interaction patterns
- Gestures, RLT/LTR, Accessibility, Responsiveness
-
Allows custom styling
- Overlays, Base Components

Drag and Drop
<div cdkDropList>
<div cdkDrag *ngFor="let card of cards">Card {{card.number}}</div>
</div>

Virtual Scroll
<cdk-virtual-scroll-viewport itemSize="4">
<img *cdkVirtualFor="let image of images" [src]="image" />
</cdk-virtual-scroll-viewport>
Ecosystem

angularconsole.com

stackblitz.com
Future



Angular Elements

Ivy Compiler




What's new in Angular 7!
By Gerard Sans
What's new in Angular 7!
Yes! We are very excited. In this talk we are going to review the main features of the latest and greatest Angular release. We will also go over some cool demos and provide top tips for a successful upgrade.
- 3,876